Image To Text Conversion With React And Tesseract.js OCR
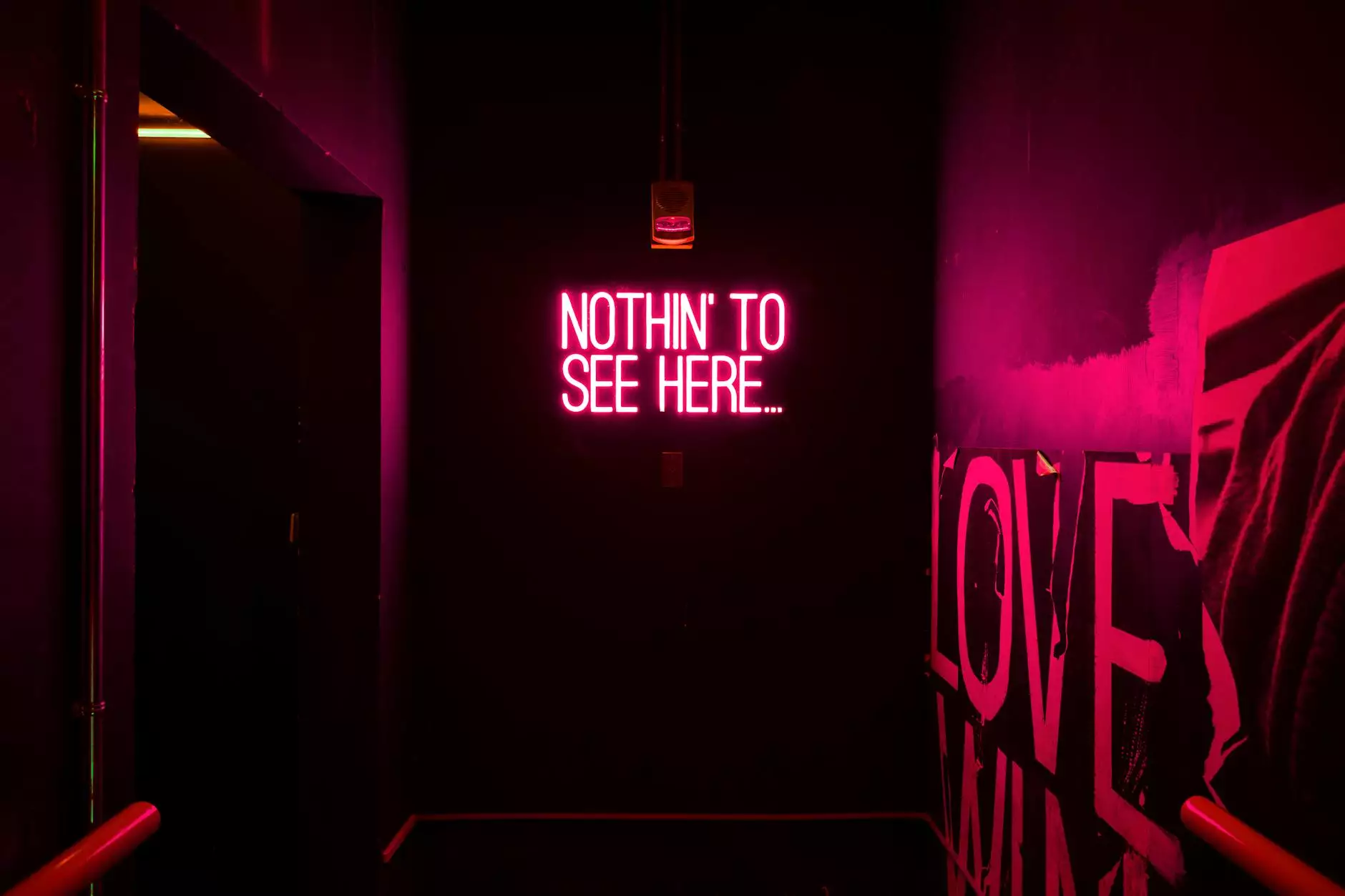
Introduction
Welcome to Shortcut Web Design's guide on image to text conversion using React and Tesseract.js OCR! If you're looking to extract text from images for various purposes, such as data analysis, document processing, or automating tasks, you've come to the right place. In this comprehensive tutorial, we will walk you through the process of utilizing React and Tesseract.js OCR to achieve accurate and efficient image to text conversion.
Why Choose Image To Text Conversion?
In today's digital age, extracting text from images has become increasingly valuable. It allows businesses and individuals to efficiently process large amounts of information contained within images, whether it's handwritten text, printed documents, or even text within complex images. By converting images to text, you can unlock numerous possibilities for automation, analysis, and enhancing overall productivity.
Understanding React
Before delving into the image to text conversion process, let's briefly touch upon React. React is a popular JavaScript library for building user interfaces, allowing developers to create reusable UI components and efficiently manage application state. Its component-based architecture, virtual DOM, and efficient rendering make it an ideal choice for developing dynamic and responsive web applications.
Introducing Tesseract.js OCR
Tesseract.js OCR is an open-source OCR (Optical Character Recognition) library based on Tesseract, an industry-leading OCR engine. By leveraging the power of Tesseract.js, developers can perform advanced text recognition on images directly within a web browser, without the need for complex server-side setups. It supports multiple languages, various image formats, and provides high accuracy and speed.
The Image To Text Conversion Process
Now, let's dive into the step-by-step process of converting images to text using React and Tesseract.js OCR:
Step 1: Setting Up the Development Environment
Before we begin, make sure you have Node.js and npm (Node Package Manager) installed on your system. Create a new React project by running the following command:
npx create-react-app image-to-text-conversionNavigate to the project directory using the terminal and install the required dependencies:
cd image-to-text-conversion npm install react-tesseract-ocrStep 2: Creating the Image To Text Component
Next, create a new file named ImageToText.js within the src directory. Import the necessary dependencies and set up the basic structure of the component:
import React from 'react'; import Tesseract from 'tesseract.js'; function ImageToText() { // Component code here }We will use this component to handle the image upload and perform the text recognition process.
Step 3: Handling Image Upload
Add the necessary code to enable image upload functionality within the ImageToText component. This can be achieved using the input element and an event handler:
import React, { useState } from 'react'; import Tesseract from 'tesseract.js'; function ImageToText() { const [image, setImage] = useState(null); const handleImageUpload = (event) => { // Image upload handling code here }; return ( ); }Users can now select an image file from their local system and trigger the image upload process.
Step 4: Performing OCR On Uploaded Image
With the uploaded image available, it's time to utilize Tesseract.js OCR to extract the text. Implement the following code within the handleImageUpload function:
import React, { useState } from 'react'; import Tesseract from 'tesseract.js'; function ImageToText() { const [image, setImage] = useState(null); const handleImageUpload = async (event) => { const file = event.target.files[0]; const { data: { text } } = await Tesseract.recognize(file); console.log(text); }; return ( ); }By using the Tesseract.recognize() method with the uploaded image, we can obtain the recognized text data, which can be further processed or displayed to the user.
Step 5: Rendering the Converted Text
Finally, let's render the converted text within the ImageToText component:
import React, { useState } from 'react'; import Tesseract from 'tesseract.js'; function ImageToText() { const [image, setImage] = useState(null); const [convertedText, setConvertedText] = useState(''); const handleImageUpload = async (event) => { const file = event.target.files[0]; const { data: { text } } = await Tesseract.recognize(file); setConvertedText(text); }; return ( {convertedText && (Converted Text:
{convertedText}
)} ); }Once the conversion is complete, the recognized text will be displayed to the user within the component.
Conclusion
Congratulations! You have successfully learned how to convert images to text using React and Tesseract.js OCR. This powerful combination allows you to unlock the potential of image data and perform various automated tasks, data analysis, and document processing. Shortcut Web Design, a leading provider of professional website development services in the field of Business and Consumer Services, offers assistance in building exceptional web applications using the latest technologies.
Take Your Image To Text Conversion to the Next Level!
If you want to optimize your business's image to text conversion process, Shortcut Web Design is here to help. Our team of highly skilled developers specializes in creating innovative and efficient solutions tailored to your specific needs. Contact us today to leverage the full potential of image recognition and take your business operations to new heights. Enhance productivity, simplify data extraction, and stay ahead of the competition with Shortcut Web Design!