How To Implement Authentication In Next.js With Auth0
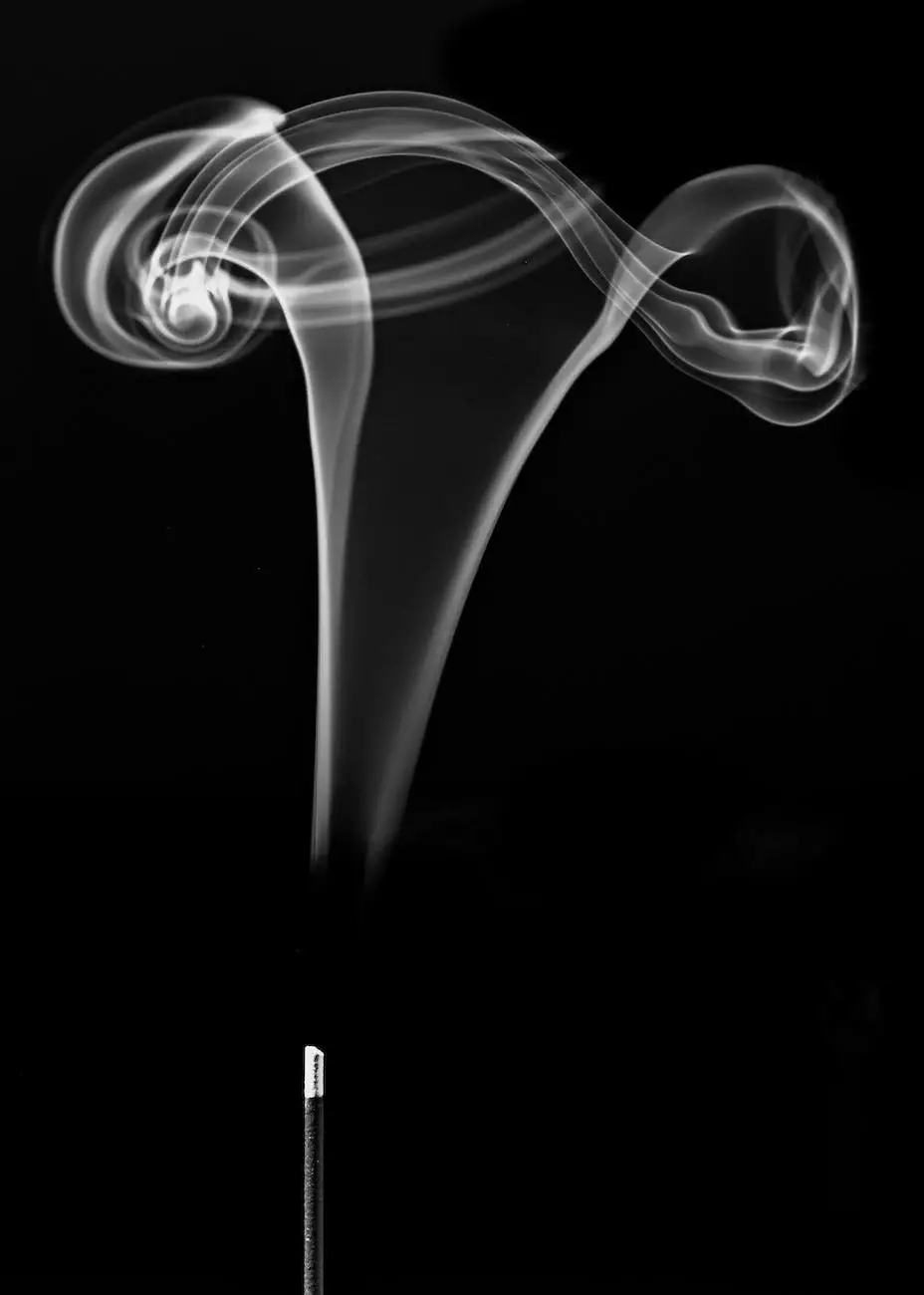
Welcome to Shortcut Web Design's comprehensive guide on how to implement authentication in Next.js with Auth0. As a leading website development company specializing in business and consumer services, we understand the importance of implementing secure and efficient authentication systems for web applications. In this guide, we will walk you through the process step-by-step, providing detailed explanations to help you successfully implement authentication in your Next.js projects.
Why Implement Authentication in Next.js?
Authentication is a critical aspect of web application development. It enables you to verify the identity of users and restrict access to certain parts of your application to authorized individuals only. By implementing authentication in Next.js, you can enhance the security of your application and ensure that user data remains protected.
Getting Started with Auth0
Auth0 is a powerful authentication and authorization platform that simplifies the implementation process. It provides robust security features, seamless integration options, and a user-friendly interface. To begin implementing authentication in Next.js with Auth0, follow these steps:
- Create an Auth0 account - Visit https://auth0.com/ and sign up for an account. It's free to get started and offers flexible pricing plans for businesses of all sizes.
- Create a new application - Once you have an Auth0 account, you need to create a new application. Navigate to the Applications tab and click on the "Create Application" button.
- Select the application type - Auth0 offers various application types, such as Single Page Applications and Regular Web Applications. Choose the one that best suits your Next.js project.
- Configure your application - Fill in the necessary details, including the application name and URLs. Take note of the provided Client ID and Domain as you will need them during the implementation process.
- Implement Auth0 in your Next.js project - Now comes the exciting part. Open your Next.js project and install the necessary dependencies. Follow the official documentation provided by Auth0 on how to integrate their SDK into your project.
Understanding the Authentication Flow
Before we dive into the implementation details, it's essential to understand the authentication flow in Next.js with Auth0. The authentication flow typically involves the following steps:
- User authentication - Users will be prompted to authenticate themselves using their preferred authentication method, such as email/password, social logins, or multi-factor authentication.
- Token generation - Once the user is authenticated successfully, Auth0 will generate an access token or ID token that represents the user's identity.
- Token verification - Next.js will receive the generated token and use Auth0's SDK to verify its authenticity and validity.
- Granting access - Once the token is verified, Next.js can grant access to the protected routes or resources within your application.
Implementing Authentication in Next.js
Now that we have a solid understanding of the authentication flow, let's start implementing authentication in Next.js. Here's a step-by-step guide to help you get started:
Step 1: Install Required Dependencies
Begin by installing the necessary dependencies for authentication in Next.js. Open your project's terminal and run the following command:
npm install @auth0/nextjs-auth0Step 2: Add Auth0 Configuration
Next, configure your Next.js application to work with Auth0. In your project's root directory, create a new file called .env.local and add the following environment variables:
AUTH0_SECRET=your_auth0_secret AUTH0_BASE_URL=your_application_base_url AUTH0_ISSUER_BASE_URL=your_auth0_issuer_base_urlReplace your_auth0_secret with your Auth0 application secret, your_application_base_url with your Next.js application base URL, and your_auth0_issuer_base_url with your Auth0 issuer base URL.
Step 3: Create Auth0 Provider
Open your Next.js _app.js file and import the necessary components:
import { Auth0Provider } from '@auth0/nextjs-auth0'; export default function App({ Component, pageProps }) { return ( ); }Make sure to replace the NEXT_PUBLIC_AUTH0_* environment variables with your Auth0 credentials.
Step 4: Implement Authentication Pages
Create two new pages in your Next.js project - api/auth/login.js and api/auth/logout.js. These pages will handle the login and logout functionality respectively. Here's an example of the login page:
import { handleAuth } from '@auth0/nextjs-auth0'; export default handleAuth();Repeat the process for the logout page, but use handleLogin() instead.
Step 5: Protect Your Routes
If you want to protect certain routes within your Next.js application, you can do so by using the withPageAuthRequired higher-order component provided by @auth0/nextjs-auth0. For example, to protect the /dashboard route, create a new file called pages/dashboard.js and add the following code:
import { withPageAuthRequired } from '@auth0/nextjs-auth0'; const Dashboard = () => { return (Welcome to your secure dashboard!
); }; export default withPageAuthRequired(Dashboard);By wrapping the Dashboard component with withPageAuthRequired, the route will only be accessible to authenticated users.
Conclusion
Congratulations! You have successfully implemented authentication in your Next.js project using Auth0. Now, your application is equipped with a robust and secure authentication system, providing users with a safe environment to access and interact with your services. Remember to continuously update and enhance your authentication system to stay ahead of potential security threats.
If you require further assistance or have any questions, don't hesitate to reach out to Shortcut Web Design, your trustful partner in website development and business and consumer services.